This VHDL project presents a simple VHDL code for a comparator which is designed and implemented in Verilog before. Full VHDL code together with test bench for the comparator is provided.
The design for the comparator based on the truth table and K-map are already presented here. There are two 2-bit inputs A and B to be compared. Three output signals are A_less_B (1 if A < B, else 0), A_equal_B (1 if A=B, else 0), and A_greater_B (1 if A>B, else 0). The minimized expressions obtained from K-Map tables for the outputs are used for VHDL coding of the comparator.
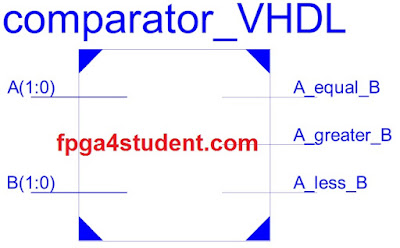
VHDL code for the comparator:
-- fpga4student.com: FPGA projects, Verilog projects, VHDL projects -- VHDL project: VHDL code for a comparator -- A comparator with 2 2-bit input A and B, and three outputs -- A less than B, A equal B, and A greater than B -- The comparator is designed by using truth table, K-Map. -- The final output expressions are used for VHDL coding library IEEE; use IEEE.std_logic_1164.all; entity comparator_VHDL is port ( A,B: in std_logic_vector(1 downto 0); -- two inputs for comparison A_less_B: out std_logic; -- '1' if A < B else '0' A_equal_B: out std_logic;-- '1' if A = B else '0' A_greater_B: out std_logic-- '1' if A > B else '0' ); end comparator_VHDL; architecture comparator_structural of comparator_VHDL is signal tmp1,tmp2,tmp3,tmp4,tmp5, tmp6, tmp7, tmp8: std_logic; -- temporary signals begin -- A_equal_B combinational logic circuit tmp1 <= A(1) xnor B(1); tmp2 <= A(0) xnor B(0); A_equal_B <= tmp1 and tmp2; -- A_less_B combinational logic circuit tmp3 <= (not A(0)) and (not A(1)) and B(0); tmp4 <= (not A(1)) and B(1); tmp5 <= (not A(0)) and B(1) and B(0); A_less_B <= tmp3 or tmp4 or tmp5; -- A_greater_B combinational logic circuit tmp6 <= (not B(0)) and (not B(1)) and A(0); tmp7 <= (not B(1)) and A(1); tmp8 <= (not B(0)) and A(1) and A(0); A_greater_B <= tmp6 or tmp7 or tmp8; end comparator_structural;
VHDL testbench code for the comparator:
-- fpga4student.com: FPGA projects, Verilog projects, VHDL projects -- VHDL project: VHDL code for a comparator -- Testbench VHDL code for comparator LIBRARY ieee; USE ieee.std_logic_1164.ALL; use ieee.numeric_std.all; ENTITY tb_comparator_VHDL IS END tb_comparator_VHDL; ARCHITECTURE behavior OF tb_comparator_VHDL IS -- Component Declaration for the comparator in VHDL COMPONENT comparator_VHDL PORT( A : IN std_logic_vector(1 downto 0); B : IN std_logic_vector(1 downto 0); A_less_B : OUT std_logic; A_equal_B : OUT std_logic; A_greater_B : OUT std_logic ); END COMPONENT; --Inputs signal A : std_logic_vector(1 downto 0) := (others => '0'); signal B : std_logic_vector(1 downto 0) := (others => '0'); --Outputs signal A_less_B : std_logic; signal A_equal_B : std_logic; signal A_greater_B : std_logic; BEGIN -- Instantiate the comparator in VHDL uut: comparator_VHDL PORT MAP ( A => A, B => B, A_less_B => A_less_B, A_equal_B => A_equal_B, A_greater_B => A_greater_B ); -- Stimulus process stim_proc: process begin -- create test cases for A_less_B for i in 0 to 3 loop A <= std_logic_vector(to_unsigned(i,2)); B <= std_logic_vector(to_unsigned(i+1,2)); wait for 20 ns; end loop; -- create test cases for A_greater_B for i in 0 to 3 loop A <= std_logic_vector(to_unsigned(i+1,2)); B <= std_logic_vector(to_unsigned(i,2)); wait for 20 ns; end loop; -- create test cases for A_equal_B for i in 0 to 3 loop A <= std_logic_vector(to_unsigned(i,2)); B <= std_logic_vector(to_unsigned(i,2)); wait for 20 ns; end loop; wait; end process; END;
Simulation waveform for the comparator in VHDL:

The simulation waveform above shows the correct operations of the comparator. Another 8-bit comparator design in VHDL is presented here.
Recommended VHDL projects:
1. What is an FPGA? How VHDL works on FPGA
2. VHDL code for FIFO memory
3. VHDL code for FIR Filter
4. VHDL code for 8-bit Microcontroller
5. VHDL code for Matrix Multiplication
6. VHDL code for Switch Tail Ring Counter
7. VHDL code for digital alarm clock on FPGA
8. VHDL code for 8-bit Comparator
9. How to load a text file into FPGA using VHDL
10. VHDL code for D Flip Flop
11. VHDL code for Full Adder
12. PWM Generator in VHDL with Variable Duty Cycle
13. VHDL code for ALU
14. VHDL code for counters with testbench
15. VHDL code for 16-bit ALU
16. Shifter Design in VHDL
17. Non-linear Lookup Table Implementation in VHDL
18. Cryptographic Coprocessor Design in VHDL
Recommended VHDL projects:
1. What is an FPGA? How VHDL works on FPGA
2. VHDL code for FIFO memory
3. VHDL code for FIR Filter
4. VHDL code for 8-bit Microcontroller
5. VHDL code for Matrix Multiplication
6. VHDL code for Switch Tail Ring Counter
7. VHDL code for digital alarm clock on FPGA
8. VHDL code for 8-bit Comparator
9. How to load a text file into FPGA using VHDL
10. VHDL code for D Flip Flop
11. VHDL code for Full Adder
12. PWM Generator in VHDL with Variable Duty Cycle
13. VHDL code for ALU
14. VHDL code for counters with testbench
15. VHDL code for 16-bit ALU
16. Shifter Design in VHDL
17. Non-linear Lookup Table Implementation in VHDL
18. Cryptographic Coprocessor Design in VHDL
20. VHDL Code for Clock Divider on FPGA
21. How to generate a clock enable signal instead of creating another clock domain
21. How to generate a clock enable signal instead of creating another clock domain
No comments:
Post a Comment